Next.js Rendering Modes
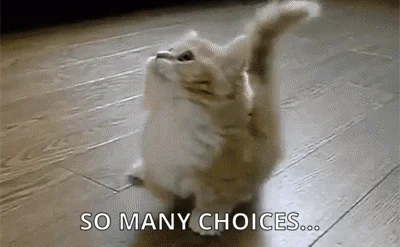
With Next.js you can choose to render your content with client side rendering, server side rendering and static rendering. You can choose one of these rendering methods for your whole application or you can choose one for the specific route. That is pretty cool!
Client Side Rendering
const [item, setItem] = useState([]);
useEffect(() => {
async function fetchData() {
const response = await fetch("");
setItem(await response.json());
}
fetchData();
}, []);
jsxNext.js uses server side rendering as a default rendering method. In this case we are not using any Next.js specific way of rendering the content. We are using useState
hook from React to set a local state for our content. That means, when the page has loaded in the browser, the page source will not include the content because it has been rendered on the client.
Server Side Rendering
export async function getServerSideProps() {
const response = await fetch("");
return {
props: {
item: await response.json(),
},
};
}
jsxWith server side rendering we can use the Next.js getServerSideProps
to fetch our content. In this way, the rendering will no longer happen on the client. The content will be server side rendered and the page will be loaded with data.
Static Rendering
export async function getStaticProps() {
const response = await fetch("");
return {
props: {
item: await response.json(),
},
};
}
jsxWith static rendering we can use the same approach as server side rendering, but instead of using getServerSideProps
we can simply use getStaticProps
. On dynamic pages such as detail pages, you will need to know the routes. You can do that with getStaticPaths
. There really is no visible difference in the browser, but you can tell the difference in the build time. Because we are using static rendering all the pages are rendered once with content. That means if you want to change the content, you will have to make a build. On the other side, with Next.js you can also use revalidate
for incremental static regeneration. Here you can define revalidate: 10
that will tell Next.js to re-generate the page when a request comes in once every 10 seconds. Holy moly that is cool!
The use case is if you want something to only change on build and want to sleep at night. This could be the home page or the detail page. The use case for incremental static regeneration could maybe also be the detail page or maybe a comment field.